You can implement some interfaces in your tool or your tool control to extends its capability in XrmToolBox. The list of interfaces can be found below
IGitHubPlugin interface
Implement this interface in PluginControlBase derived class
Implements this interface to add a menu in XrmToolBox when your plugin is opened and visible. This menu allows to redirect the user to the Issues
page of the specified GitHub repository.
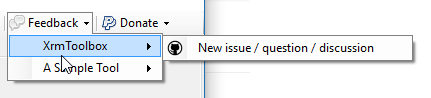
#region IGitHubPlugin implementation
public string RepositoryName => "GithubRepositoryName";
public string UserName => "GithubUserName";
#endregion IGitHubPlugin implementation
IPayPalPlugin interface
Implement this interface in PluginControlBase and/or in PluginBase derived class
If you implement the interface in the PluginControlBase class, it adds a menu in XrmToolBox when your plugin is opened and visible
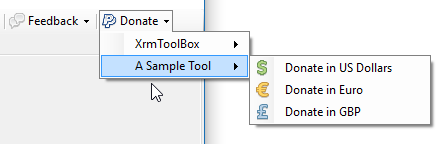
if you implement the interface in the PluginBase class, it allows your tool to be listed in tools that accept donation when a user click on a general link to make a donation (coming in February 2021).
#region IPayPalPlugin implementation
public string DonationDescription => "paypal description";
public string EmailAccount => "paypal@paypal.com";
#endregion IPayPalPlugin implementation
IHelpPlugin interface
Implement this interface in PluginControlBase derived class
Implements this interface to add a menu in XrmToolBox when your plugin is opened and visible. This menu allows to redirect the user to a web help page.
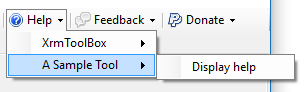
#region IHelpPlugin implementation
public string HelpUrl => "http://www.google.com";
#endregion IHelpPlugin implementation
IStatusBarMessenger interface
Implement this interface in PluginControlBase derived class
Implement this interface to allow your plugin to send process progress information to XrmToolBox main application. This information can be displayed as text, progress bar or both in the XrmToolBox status bar.
The event needs implementing in your derived class too, as shown.
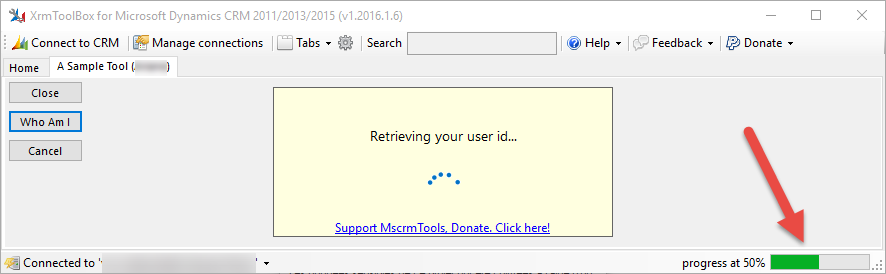
public event EventHandler<StatusBarMessageEventArgs> SendMessageToStatusBar;
private void SomeMethod()
{
// If you want to notify the user of progress, through the
// status bar, use the following method
SendMessageToStatusBar?.Invoke(this, new StatusBarMessageEventArgs(50, "progress at 50%"));
}
private void SomeFinalMethod()
{
// If you want to clear the message in the status bar use a blank argument like below
SendMessageToStatusBar(this, new StatusBarMessageEventArgs(string.Empty));
}
IDuplicatableTool interface
Implement this interface in PluginControlBase derived class
Implement this interface to allow your tool to be duplicated when right clicking on its tab header. You can pass state from the source tool instance to the new opened instance
#region IDuplicatableTool implementation
public event EventHandler<DuplicateToolArgs> DuplicateRequested;
public void ApplyState(object state)
{
txtState.Text = state.ToString();
}
public object GetState()
{
return txtState.Text;
}
private void tsbDuplicate_Click(object sender, EventArgs e)
{
DuplicateRequested?.Invoke(this, new DuplicateToolArgs("My custom state", true));
}
#endregion IDuplicatableTool implementation
IShortcutReceiver interface
Implement this interface in PluginControlBase derived class
Implements this interface to allow your tool to receive user keyboard inputs
#region Shortcut Receiver implementation
public void ReceiveKeyDownShortcut(KeyEventArgs e)
{
// MessageBox.Show("A KeyDown event was received!");
}
public void ReceiveKeyPressShortcut(KeyPressEventArgs e)
{
// MessageBox.Show("A KeyPress event was received!");
}
public void ReceiveKeyUpShortcut(KeyEventArgs e)
{
//MessageBox.Show("A KeyUp event was received!");
}
public void ReceivePreviewKeyDownShortcut(PreviewKeyDownEventArgs e)
{
//MessageBox.Show("A PreviewKeyDown event was received!");
}
public void ReceiveShortcut(KeyEventArgs e)
{
MessageBox.Show(e.ToString());
}
#endregion Shortcut Receiver implementation
IMessageBusHost interface
Implement this interface in PluginControlBase derived class
Implement this interface to allow cross tools communication. See documentation from Jonas Rapp
IAboutPlugin interface
Implement this interface in PluginControlBase derived class
Implements this interface to display an About menu for your tool in XrmToolBox Help menu. Do whatever you want when this menu is clicked
#region IAboutPlugin implementation
public void ShowAboutDialog()
{
MessageBox.Show(@"This is a sample tool", @"About Sample Tool", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
#endregion IAboutPlugin implementation
ISettingsPlugin interface
Implement this interface in PluginControlBase derived class
Implements this interface to add a Settings menu for your tool in XrmToolBox Configuration menu
#region ISettingsPlugin implementation
public void ShowSettings()
{
MessageBox.Show(@"Settings should be displayed instead of this dialog");
}
#endregion ISettingsPlugin implementation
IPrivatePlugin interface
Implement this interface in PluginControlBase derived class
Implements this interface to instruct XrmToolBox to not report tool opening to Application Insights
INoConnectionRequired interface
Implement this interface in PluginBase derived class
Implement this interface if your plugin does not need a connection before being opened.
ICompanion interface
Implement this interface in PluginControlBase derived class
Implements this interface to instruct XrmToolBox to display the tool as a companion. That means the tool will be docked on Left or Right side of XrmToolBox depending on how you implement the interface.
Your tool must have a limited width since the purpose of the companion is to be always displayed alongside other tools in XrmToolBox
#region ICompanion implementation
public LeftOrRight GetPosition()
{
return LeftOrRight.Left;
}
#endregion ICompanion implementation