Many XrmToolBox Tools require a only single connection to a Dynamics CRM tenant and one OrganizationService instance. For these Tools, the base class PluginControlBase should provide all of the properties and methods for working with your Dynamics CRM instance.
Other XrmToolBox Tools require connections to more than one Dynamics CRM instance. For example, the Document Templates Mover plugin will copy Document Templates from a Source environment to a Target environment:
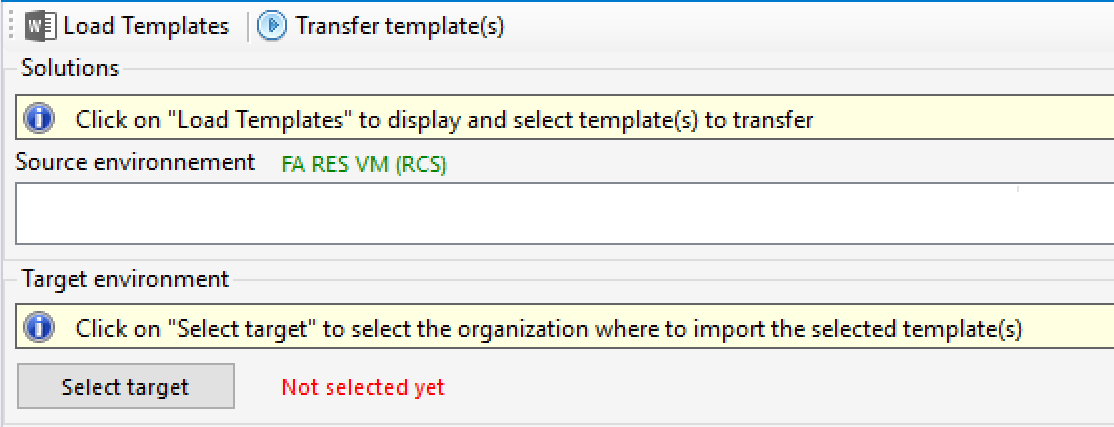
( View Tool project On GitHub: https://github.com/MscrmTools/MscrmTools.DocumentTemplatesMover )
The MultipleConnectionsPluginControlBase class provides several additional built in Properties and Methods that provide a standard method for connecting to and managing multiple connections for a single XrmToolBox Tool.
Because this class derives from PluginControlBase class, all properties and methods of this base class will also be available to your new XrmToolBox tool. For details on the PluginControlBase class, see the following article: PluginControlBase base class
Properties
AdditionalConnectionDetails
ObservableCollection AdditionalConnectionDetails { get; set; }
The AdditionalConnectionDetails property offers a collection of ConnectionDetail objects managed by the base class. These ConnectionDetail objects are only available within the scope of your XrmToolBox tool and are not accessible by other loaded Tools as with the Service and ConnectionDetail properties provided by the PluginControlBase class.
You can interact with the AdditionalConnectionDetails collection objects as you would the ConnectionDetail object provided by the PluginControlBase class. For example, calling a WhoAmIRequest for each Dynamics CRM instance in the collection:
foreach (var detail in AdditionalConnectionDetails)
{
var resp = detail.GetCrmServiceClient().Execute(new WhoAmIRequest()) as WhoAmIResponse;
}
You can add and remove items to this collection directly. For example, to remove an organization from the list of organizations, simply remove an item from the property AdditionalConnectionDetails
var toRemove = AdditionalConnectionDetails.FirstOrDefault(c => c.ConnectionName == "My Connection");
if (toRemove != null)
{
AdditionalConnectionDetails.Remove(toRemove);
}
When the ConnectionDetail object is added or removed, the ConnectionDetailsUpdated method is called with an Action value of “Add” or “Remove”.
Methods
ConnectionDetailsUpdated
abstract void ConnectionDetailsUpdated(NotifyCollectionChangedEventArgs e);
The ConnectionDetailsUpdated method is an abstract method meant to be overridden by the your derived XrmToolBox tool class. This method is called by the base class each time the collection is updated.
The NotifyCollectionChangedEventArgs arguments provided will allow you to distinguish whether a new connection has been Added or Removed from the AdditionalConnectionDetails collection.
This method will be called whether you update the collection directly through the Add() or Remove() methods, or by calling AddAdditionalOrganization() or RemoveAdditionalOrganization() .
AddAdditionalOrganization
void AddAdditionalOrganization();
The AddAdditionalOrganization method allows you to connect to a Dynamics CRM instance that will be available to only your XrmToolBox Tool. When called, this method will launch the common XrmToolBox Connect dialog and allow the user to connect to an organization.
Each time this method is called and the user makes a successful connection, the new ConnectionDetail object will be added to the AdditionalConnectionDetails collection.
When the ConnectionDetail object is added, the ConnectionDetailsUpdated method is called with an Action value of “Add”. To connect to an additional organization, you can simply call the method AddAdditionalOrganization . For example, assume that we have an XrmolBox Tool with a button named tsbAddTargetOrg :
private void tsbAddTargetOrg_Click(object sender, EventArgs e)
{
AddAdditionalOrganization();
}
NOTE: Currently, it is not possible to select multiple organizations in one single operation. You need to repeat the action to add multiple additional organizations.
|
RemoveAdditionalOrganization
void RemoveAdditionalOrganization(ConnectionDetail detail);
The RemoveAdditionalOrganization method allows you to remove a ConnectionDetail object from the AdditionalConnectionDetails collection. If you are changing a connection or disconnecting from a Dynamics CRM instance, you can call this method to update the AdditionalConnectionDetails collection.
When the ConnectionDetail object is removed, the ConnectionDetailsUpdated method is called with an Action value of “Remove”.
Sample Project
You can download the sample XrmToolBox Tool project that implements some of the methods described in this article.
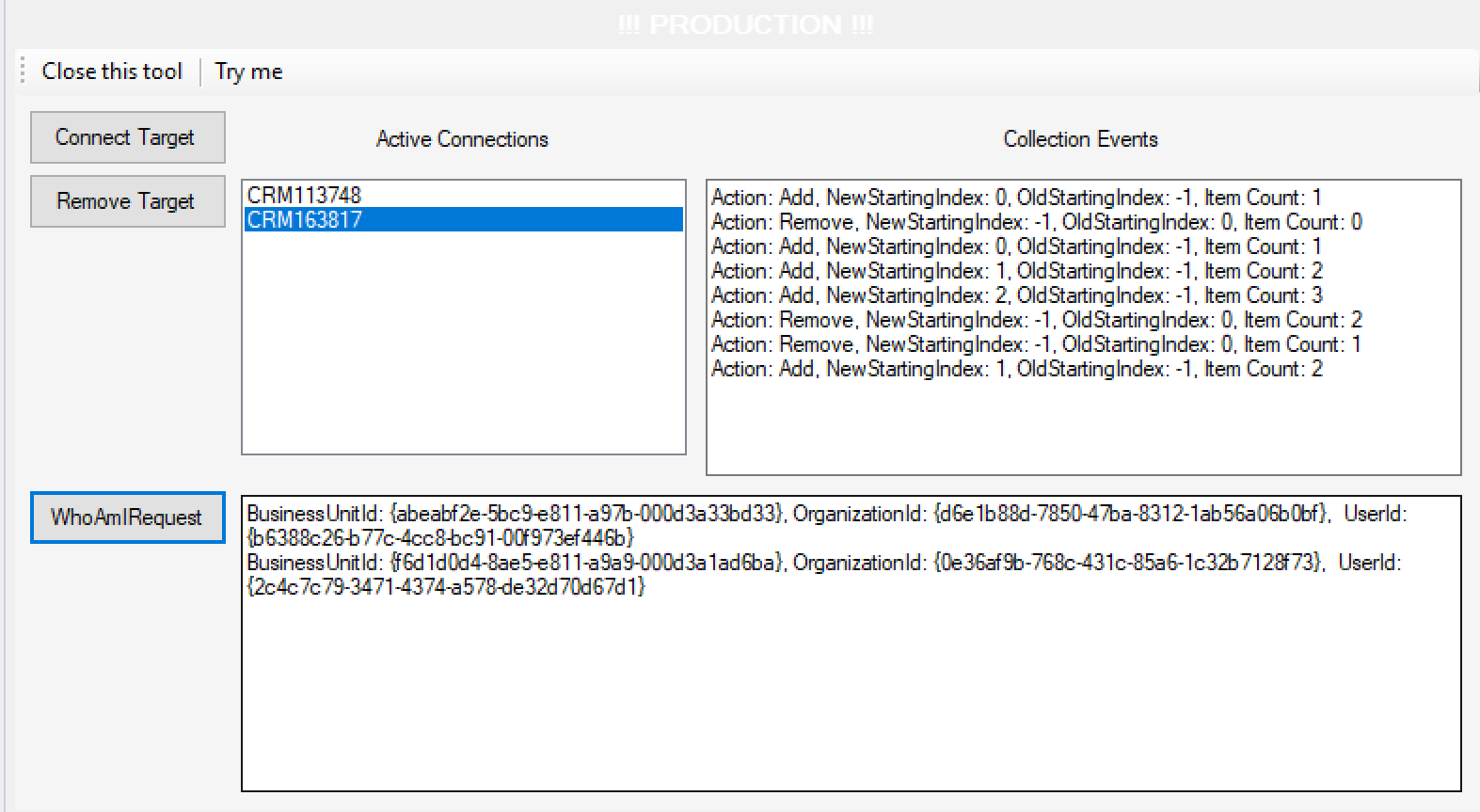
This sample project contains an XrmToolBox Tool deriving from the MultipleConnectionsPluginControlBase base class. The sample Tool will allow you to connect to CRM one or more times and will display the list of ConnectionDetail objects in the AdditionalConnectionDetails collection. The Connection Events section shows the log of each call to the ConnectionDetailsUpdated method, displaying the type of action and some additional details about the event. If you select one of the available connections from the Active Connections list, you can remove it from the AdditionalConnectionDetails collection and observe the Remove messages. Lastly, the WhoAmIRequest button executes a WhoAmIRequest for each item in collection and will log the results in the text box to the right.
Download the sample project as a zip file here: Sample Project: MultipleConnectionsPluginControlBase base class.