This class is a base class to implement an XrmToolBox plugin control. It comes with several built in Properties and Methods that will help you create an optimized plugin.
This base class only allows one OrganizationService to be used. If you need multiple OrganizationService (for example, to transfer some data form a Microsoft Dynamics 365 CE organization to another one), please use base class MultipleConnectionsPluginControlBase
. This is a child class of PluginControlBase
class so all information on this page still applies to MultipleConnectionsPluginControlBase
.
DO NOT USE Early Bound Entities or your plugin code logic. That will make a conflict with other plugins and make XrmToolBox unable to connect to any organization. It will also lead to bad reputation for your plugin!
Working with Dynamics 365 CE OrganizationService
Access the organization service
To access the organization service, just use the property Service
in your user control.
Service.Execute(new WhoAmIRequest());
Ensure service is available
When a user opens your plugin, he can open it without being connected to an organization. So, it is important that each time a method requires the organization service to be initialized, you use the method ExecuteMethod
. This way, the user will be prompted to select an organization to connect to before your method is executed.
private void MyPluginControl_Load(object sender, EventArgs e)
{
ExecuteMethod(WhoAmI);
}
private void WhoAmI()
{
Service.Execute(new WhoAmIRequest());
}
Organization service change
A user can decide to change the connection of an already open plugin. In this case, Service property is automatically updated. But sometimes, you may want get more information about this change or perform some action after this change (like reloading some data for the newly connected organization). You can override the method UpdateConnection
to get more information or perform some action.
public override void UpdateConnection(IOrganizationService newService, ConnectionDetail detail, string actionName, object parameter)
{
// Add some actions here
base.UpdateConnection(newService, detail, actionName, parameter);
}
The method parameters are:
- newService : the OrganizationService for the organization connected
- detail : the détails of the connection selected
- actionName : this parameter may contain some information about the action that asked for an OrganizationService change
- parameter : this parameter may contain additional parameter passed when the OrganizationService change has been requested
Write your plugin logic asynchronously
Your code logic should perform work asynchronously to avoid freezing the application UI. In order to simplify multithreading logic, the base provides a method with multiple signatures: WorkAsync
This method is an abstraction of BackgroundWorker that can take an argument, do some work on another thread, report progress and get back to main thread to finalize the process.
WorkAsync(new WorkAsyncInfo
{
Message = "Retrieving your user id...",
Work = (w, e) =>
{
// This code is executed in another thread
var request = new WhoAmIRequest();
var response = (WhoAmIResponse)Service.Execute(request);
w.ReportProgress(-1, "I have found the user id");
e.Result = response.UserId;
},
ProgressChanged = e =>
{
// it will display "I have found the user id" in this example
SetWorkingMessage(e.UserState.ToString());
},
PostWorkCallBack = e =>
{
// This code is executed in the main thread
MessageBox.Show($"You are {(Guid) e.Result}");
},
AsyncArgument = null,
// Progress information panel size
MessageWidth = 340,
MessageHeight = 150
});
Error handling
From version 1.2022.2.54 of XrmToolBox, a new feature to show error message in a common way, easily to be used, and the same way of all tools.
It will try to find the 'actual error' and show it to make it easier for the user to fix it. If the user needs more info, you can show details, and may be copying or even create a new issue (if the tool has IGitHubPlugin
). To the issue you may also add more info.
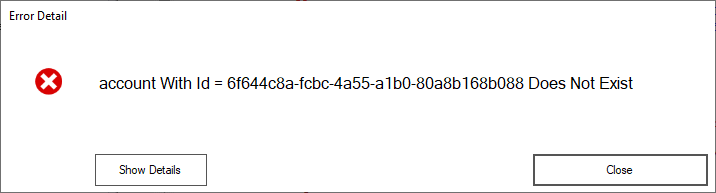
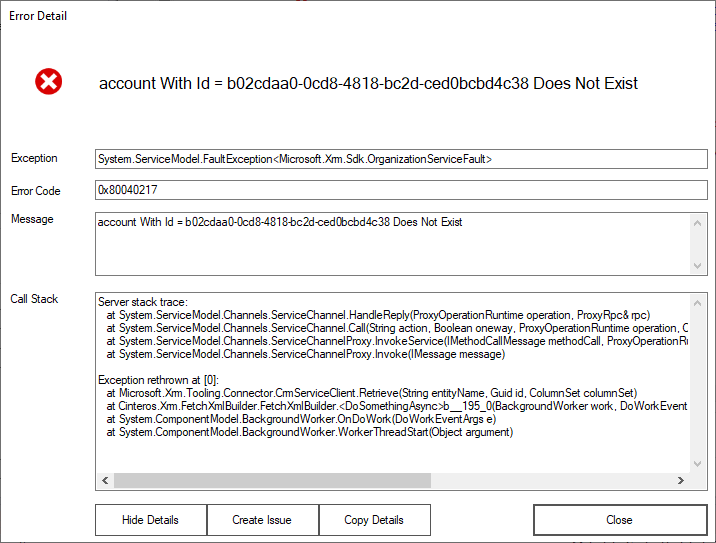
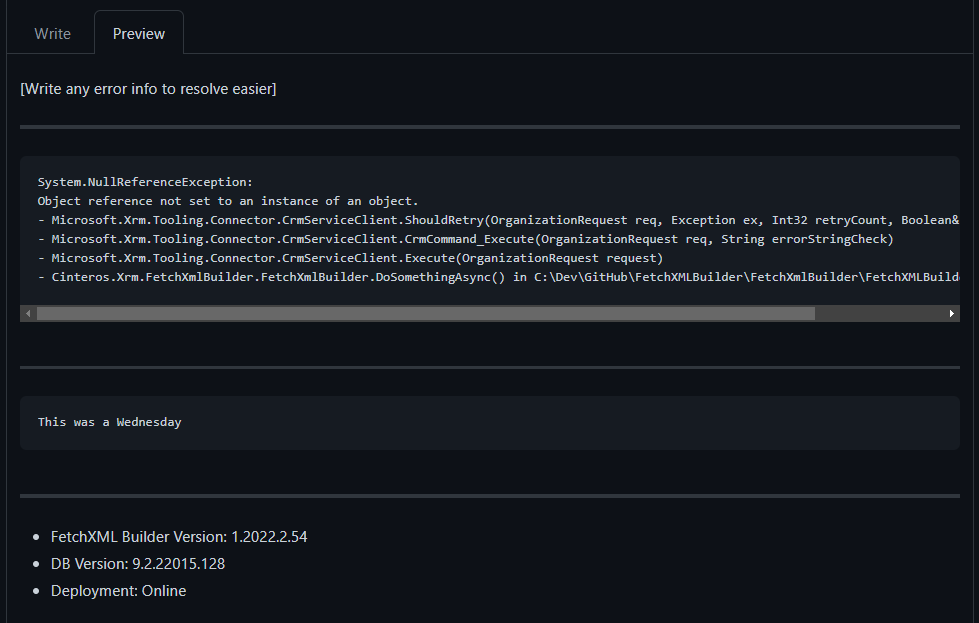
Sample simple call:
WorkAsync(new WorkAsyncInfo
{
Message = "Something...",
Work = (work, args) =>
{
args.Result = Service.Retrieve("account", Guid.NewGuid(), new ColumnSet("name"));
},
PostWorkCallBack = args =>
{
if (args.Error != null)
{
ShowErrorDialog(args.Error);
return;
}
var someresult = args.Result as OrganizationResponse;
}
});
Sample advanced call:
try
{
var result = Service.Execute(request);
}
catch (FaultException<OrganizationServiceFault>) { }
catch (Exception ex)
{
ShowErrorDialog(ex, "Request failed", $"This was a {DateTime.Now.DayOfWeek}", true);
}
Calling signature:
public void ShowErrorDialog(Exception exception, string heading = null, string extrainfo = null, bool allownewissue = true)
Logging
Logging mechanism is automatically embedded in PluginControlBase
class. You just have to use dedicated methods to perform logging.
// Log information, warning and error messages
LogInfo("An information message");
LogWarning("A warning message");
LogError("An error message");
// Opens the log file
OpenLogFile();
Log file is stored in the storage folder of XrmToolBox in the folder Logs
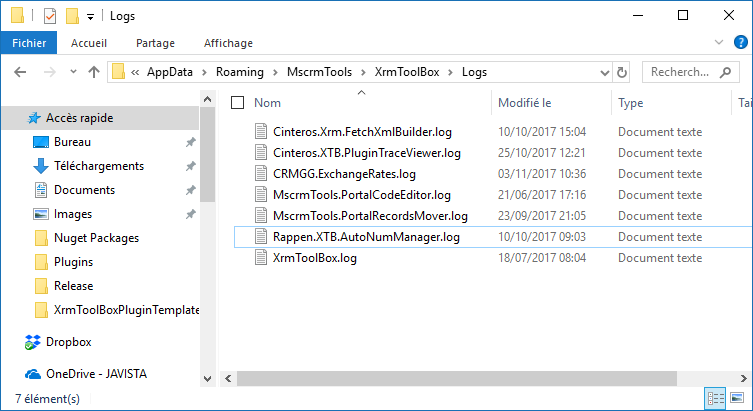
Note that the log file automatically include date time and connection name (see example below)
2017-06-21 05:16:45.944 MyOrganization Error Unable to load code items: Please ensure you are targeting an organization linked to a Microsoft Portal (not a legacy Adxstudio one)
Notification
You can display notification on your plugin to provide additional information or warning or error to the user.
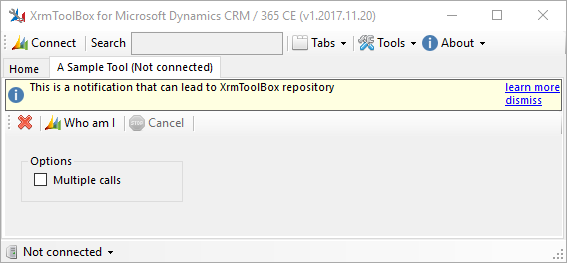
Only one notification can be displayed. The Uri, if not null, display a "more information" link that can be clicked to navigate to the Uri. The height of the notification is 32 px by default but you can adjust it, if needed.
ShowInfoNotification("You can visit XrmToolBox portal", new Uri("https://www.xrmtoolbox.com"), 32);
ShowWarningNotification("You can visit XrmToolBox portal", new Uri("https://www.xrmtoolbox.com"), 32);
ShowErrorNotification("You can visit XrmToolBox portal", new Uri("https://www.xrmtoolbox.com"), 32);
// Call this method to hide the notification
HideNotification();
Extra features
You can implement extra features by implementing XrmToolBox interfaces in your code